In this post I will describe how to test terraform with OCI, for instance from your windows laptop.
This is not intended for production server.
Install OCI cli on your desktop or server
On your laptop (or on your server) install OCI cli in order to be able to test OCI API.
Add an api key to your OCI user
Navigate to Identity > Domains > ‘Your domain’ > Users > ‘Your user’ > API Keys
Generate an RSA key with .pem extension
Now generate an RSA private key (it’s important to generate and RSA private key because the OCI terraform waits for an RSA key as explained here).
It’s a good idea to set a passphrase to your private key.
# If you haven't already, create a .oci directory to store the credentials. For example: mkdir %HOMEDRIVE%%HOMEPATH%\.oci # Generate your private key with a pass phrase (recommended) openssl genrsa -out %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key.pem -aes128 -passout stdin 4096 # If you do not want a pass phrase: # openssl genrsa -out %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key.pem 4096 # Generate the public key from the private key: openssl rsa -pubout -in %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key.pem -out %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key_public.pem
C:\Users\nfourniol>openssl genrsa -out %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key.pem -aes128 -passout stdin 4096
Generating RSA private key, 4096 bit long modulus (2 primes)
..................................................................................................................................++++
.....................................................................................................................................................................................................................++++
e is 65537 (0x010001)
Enter pass phrase for C:\Users\nfourniol\.oci\oci_api_key.pem:
Verifying - Enter pass phrase for C:\Users\nfourniol\.oci\oci_api_key.pem:
C:\Users\nfourniol>openssl rsa -pubout -in %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key.pem -out %HOMEDRIVE%%HOMEPATH%\.oci\oci_api_key_public.pem
Enter pass phrase for C:\Users\nfourniol\.oci\oci_api_key.pem:
writing RSA key
Upload the generated public key
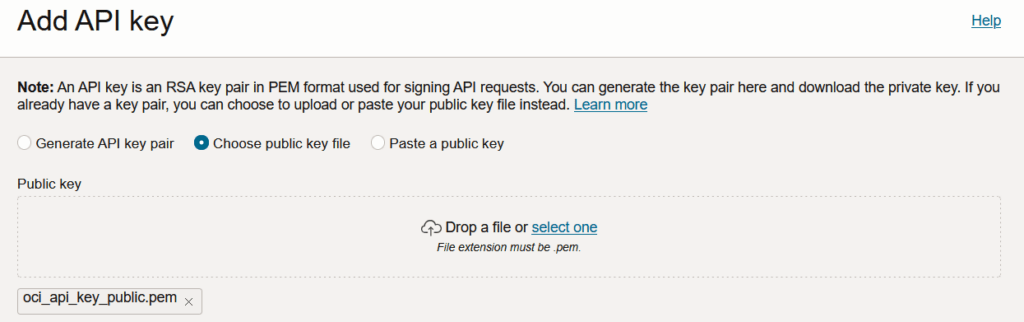
Now you validate and OCI show you the configuration file content that you set inside C:\\Users\youruser\.oci\config
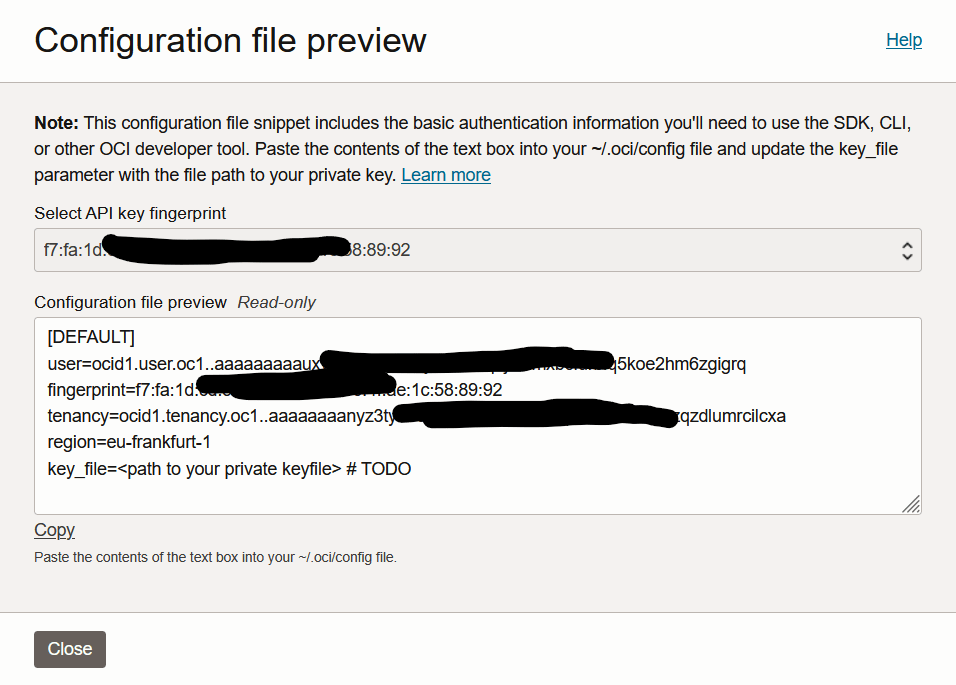
If you set a pass phrase, add the following line inside your config file:
pass_phrase=<your pass phrase>
Now you config file is something like:
[DEFAULT]
user=ocid1.user.oc1..aaaaaaaaauxXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
fingerprint=f7:fa:1d:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:92
tenancy=ocid1.tenancy.oc1..aaaaaaaaXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
region=eu-frankfurt-1
key_file=C:\\Users\\nfourniol\\.oci\\oci_api_key.pem
pass_phrase=<your pass phrase>
Test you config file with OCI cli
oci compute instance list --compartment-id ocid1.tenancy.oc1..XXXXXXXXXXXXXXXX_your_compartment_idXXXXX
C:\Users\nfourniol\Documents\dev\oci_automation\terraform-novrh\list_instances>oci compute instance list --compartment-id ocid1.tenancy.oc1..XXXXXXXXXXXXXXXX_your_compartment_idXXXXX
WARNING: Permissions on C:\\Users\\nfourniol\\.oci\\oci_api_key.pem are too open.
The following users / groups have permissions to the file and should not: NT AUTHORITY\Système S-1-5-5-0-1569054 NT AUTHORITY\Système.
To fix this please try executing the following command:
oci setup repair-file-permissions --file C:\\Users\\nfourniol\\.oci\\oci_api_key.pem
Alternatively to hide this warning, you may set an environment variable; Windows and PowerShell commands follow:
SET OCI_CLI_SUPPRESS_FILE_PERMISSIONS_WARNING=True
$Env:OCI_CLI_SUPPRESS_FILE_PERMISSIONS_WARNING="True"
If you get the error “permissions on your key are too open” then you should verify:
- in case you are on windows, the Properties > Security on your private key should have only users “System”, “<yourself>”, and “Administrators”. You can remove other users
- in case you are on linux, ensure chmod 600 on your private key
Now you can execute the oci command without error.
Terraform test
Install Terraform
On windows laptop you can use chocolatey to install Terraform.
Create a simple terraform project to list all instances from a compartment
Create a list_instances.tf file:
# Configuration of the OCI provider provider "oci" { config_file_profile = "DEFAULT" # Ensure it corresponds to your OCI cli profile } # Data source to get compute instances data "oci_core_instances" "all_instances" { compartment_id = var.compartment_id } # Output to display instances names output "instance_names" { value = [for instance in data.oci_core_instances.all_instances.instances : instance.display_name] } # Variable for compartment_id variable "compartment_id" { type = string description = "OCID du compartiment contenant les instances" }
Create a terraform.tfvars file:
compartment_id = "ocid1.compartment.oc1..XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" # replace with your compartment_id
Now execute terraform init:
C:\Users\nfourniol\Documents\dev\oci_automation\terraform-novrh\list_instances> terraform init
Initializing the backend...
Initializing provider plugins...
- Finding latest version of hashicorp/oci...
- Installing hashicorp/oci v6.30.0...
- Installed hashicorp/oci v6.30.0 (signed by HashiCorp)
Terraform has created a lock file .terraform.lock.hcl to record the provider
selections it made above. Include this file in your version control repository
so that Terraform can guarantee to make the same selections by default when
you run "terraform init" in the future.
╷
│ Warning: Additional provider information from registry
│
│ The remote registry returned warnings for registry.terraform.io/hashicorp/oci:
│ - For users on Terraform 0.13 or greater, this provider has moved to oracle/oci. Please update your source in
│ required_providers.
╵
Terraform has been successfully initialized!
You may now begin working with Terraform. Try running "terraform plan" to see
any changes that are required for your infrastructure. All Terraform commands
should now work.
If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working directory. If you forget, other
commands will detect it and remind you to do so if necessary.
Terraform init downloads the provider.
Then you can execute terraform plan and terraform apply.
Terraform apply will output values to the terraform state file terraform.tfstate.
Troubleshooting
ERROR: Terraform OCI provider error encounter bad configuration: did not find a proper configuration for private key
This error happen if your private key is not an RSA key.
Leave a Reply